Master C++ data structures with hands-on learning, focusing on linked lists, stacks, queues, and trees for efficient real-world applications.
Master C++ data structures with hands-on learning, focusing on linked lists, stacks, queues, and trees for efficient real-world applications.
This comprehensive course explores linear data structures and trees in C++, designed for programmers with a foundation in C++ basics. The hands-on, video-free approach provides a practical learning experience through runnable code examples and immediate feedback. Students will gain expertise in implementing various linked list structures (singly, doubly, circular), working with stacks and queues, and understanding tree data structures including binary search trees and self-balancing trees. The course emphasizes practical implementation skills that can be transferred to any programming language. Through progressive exercises and coding challenges, learners will develop the ability to select appropriate data structures for different problems, implement them efficiently, and understand the trade-offs between different implementation approaches.
Instructors:
English
What you'll learn
Implement various linked list structures including singly, doubly, and circular linked lists
Design and use iterators for traversing data structures efficiently
Build and utilize stack data structures for Last-In-First-Out operations
Implement queue-based structures including standard queues, priority queues, and deques
Create binary trees and binary search trees with appropriate operations
Apply different tree traversal methods including in-order, pre-order, and post-order
Skills you'll gain
This course includes:
16 Hours PreRecorded video
20 app items
Access on Mobile, Tablet, Desktop
FullTime access
Shareable certificate
Top companies offer this course to their employees
Top companies provide this course to enhance their employees' skills, ensuring they excel in handling complex projects and drive organizational success.
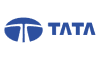
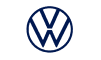
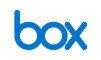

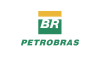
There are 4 modules in this course
This course provides a comprehensive exploration of data structures in C++, focusing on both linear structures and trees. The curriculum is structured across four modules that progressively build on each other. Students begin with the fundamentals of the List Abstract Data Type (ADT), learning various linked list implementations including singly linked, doubly linked, and circular linked lists, as well as working with iterators. The second module covers stacks and queues, introducing Last-In-First-Out (LIFO) and First-In-First-Out (FIFO) data structures, along with priority queues and double-ended queues (deques). The third module transitions to non-linear structures with the Tree ADT, covering binary trees, binary search trees, and traversal algorithms. The final module explores advanced self-balancing tree structures including AVL trees, Red-Black trees, and B-trees. Throughout the course, students engage with hands-on coding exercises that reinforce theoretical concepts with practical implementation.
List ADT
Module 1 · 4 Hours to complete
Stacks and Queues ADT
Module 2 · 4 Hours to complete
Tree ADT
Module 3 · 4 Hours to complete
Self-Balancing Trees
Module 4 · 3 Hours to complete
Fee Structure
Instructor
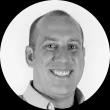
3 Courses
Experienced Educator and Director of Content at Codio
Patrick Ester, the Director of Content at Codio, brings a wealth of teaching experience to his role in educational technology. With a decade of classroom experience, Ester specialized in integrating computer-based activities into the curriculum and teaching coding to students. His expertise extends to leading workshops on physical computing, demonstrating his commitment to hands-on, practical technology education. At Codio, Ester leverages his background to develop comprehensive Python programming courses, including "Python Programming: Basic Skills," "Python Programming: Intermediate Concepts," and "Python Programming: Object-Oriented Design." His transition from classroom teaching to directing content for an educational technology platform showcases his ability to apply practical teaching experience to the development of online learning resources, particularly in the field of computer science and programming education.
Testimonials
Testimonials and success stories are a testament to the quality of this program and its impact on your career and learning journey. Be the first to help others make an informed decision by sharing your review of the course.
Frequently asked questions
Below are some of the most commonly asked questions about this course. We aim to provide clear and concise answers to help you better understand the course content, structure, and any other relevant information. If you have any additional questions or if your question is not listed here, please don't hesitate to reach out to our support team for further assistance.